The process for creating a Team in the API is quite simple, but there are a few different ways you may go about it depending on your specific case. Below, we'll go through the general options and process for creating a Team, regardless of where you're starting from.
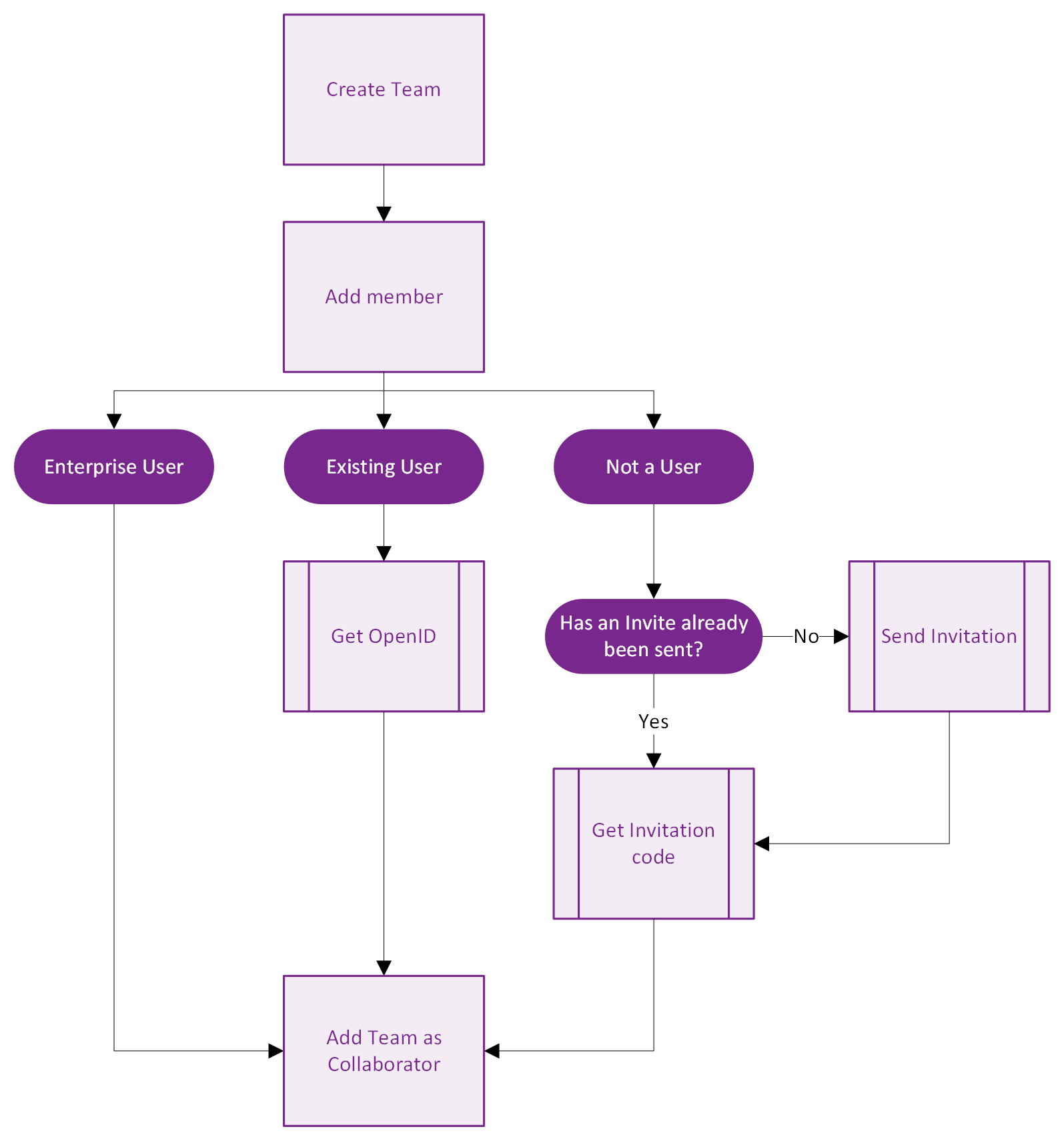
Create Team
First, create the Team itself. This means just the Team name and the affiliate code (brandCode
) it belongs to. There won't yet be any members in the Team.
POST
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/teams
{ "name": "string", "brandCode": "string", "logo": null }
{ "name": "string", "brandCode": "string", "logo": null, "active": true, "tenant": "string", "owner": "string", "type": "sidedrawer", "createdAt": "2024-08-20T13:42:14.213Z", "updatedAt": "2024-08-20T13:42:14.213Z", "id": "string" }
Adding a member
Now that the Team has been created, you can add the members who should belong to this Team.
To add a Team member, you will need one of the following identifiers:
- OpenID (
accountOpenId
) - Invitation code (
invitationCode
) - Federation ID (
federationId
) – this parameter is only for enterprise users; ignore if not an enterprise user
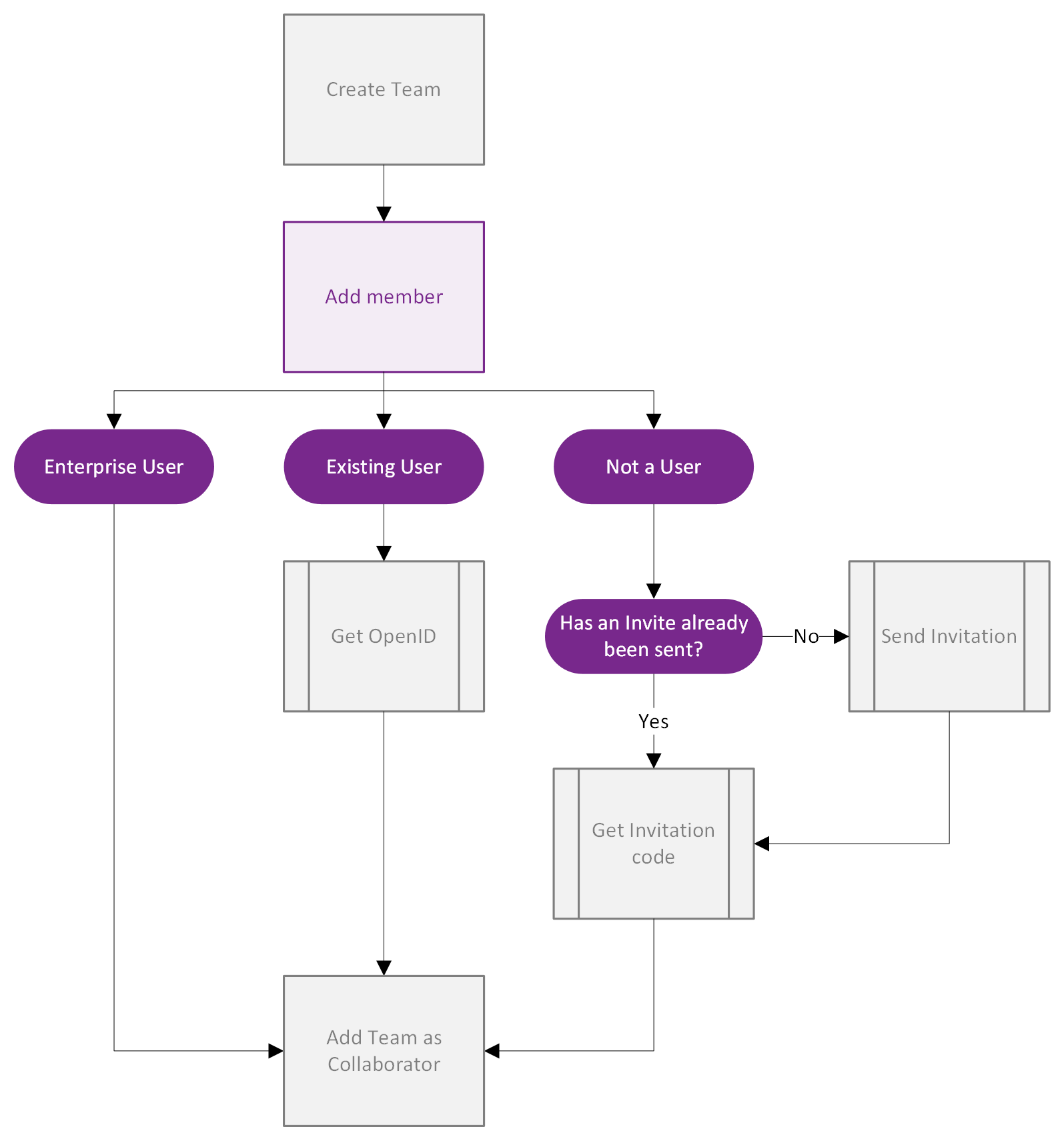
Existing User
If you would like to add a Team member who is already a SideDrawer User, you will use their OpenID. If you don't have their OpenID, you can use the following endpoint:
Get OpenID
Find a User's OpenID given their username (their email address).
GET
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/username/{username}/open-id
If you're only adding existing Users to your Team, jump ahead. Otherwise, continue on below to see how to add someone who is not yet a SideDrawer User.
Not a User
To add a member who is not an existing User, you will use their SideDrawer Invitation code. If you haven't yet sent them an Invitation, send one using the endpoint below.
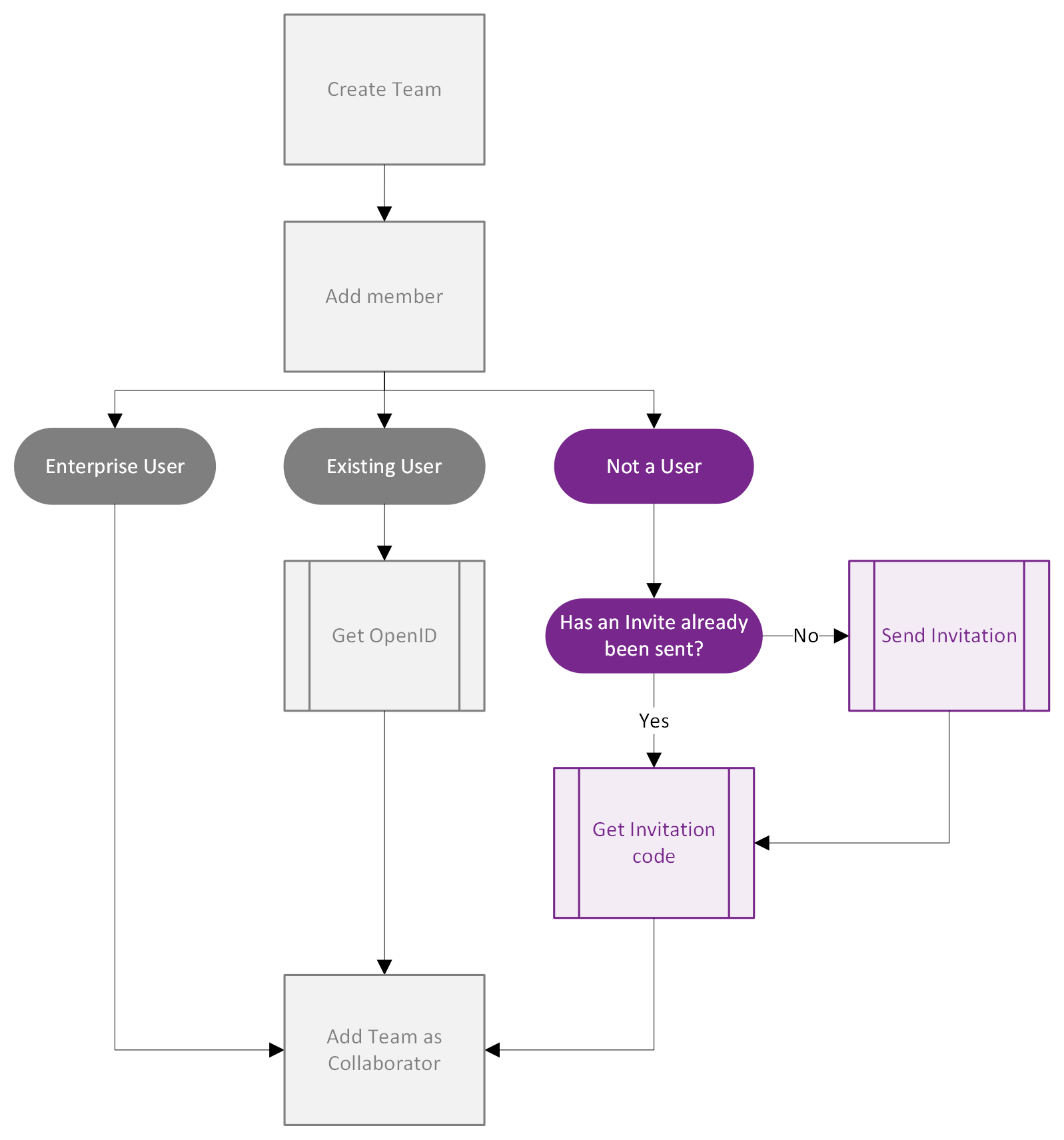
Send Invitation
POST
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/invitations
{ "firstName": "string", "lastName": "string", "email": "string", "phone": "string", "brandCode": "string", "region": "string", "emailTemplate": "string" }
Get Invitation Code
If you've already sent this person an Invitation to SideDrawer, you can use this endpoint to find the Invitation code that was sent to their email:
GET
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/invitations?email={email}
Add member
Now that you have an OpenID and/or Invitation code, call the "add Team member" endpoint using the appropriate identifier.
For existing User:
POST
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/teams/team-id/{teamId}/member
{ "accountOpenId": "string", "defaultTeam": false }
{ "active": true, "team": { "name": "string", "brandCode": "string", "logo": null, "active": true, "tenant": "string", "owner": "string", "type": "sidedrawer", "createdAt": "2024-08-20T13:42:14.213Z", "updatedAt": "2024-08-20T13:42:14.213Z", "id": "string" }, "member": "string", /*this is the member's OpenID*/ "createdAt": "2024-08-20T13:58:08.260Z", "updatedAt": "2024-08-20T13:58:08.260Z", "id": "string" }
For Invitee:
POST
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/teams/team-id/{teamId}/member
{ "invitationCode": "string" }
{ "active": true, "team": { "name": "string", "brandCode": "string", "logo": null, "active": true, "tenant": "string", "owner": "string", "type": "sidedrawer", "createdAt": "2024-08-20T13:42:14.213Z", "updatedAt": "2024-08-20T13:42:14.213Z", "id": "string" }, "invitationCode": "string", "createdAt": "2024-08-20T13:42:36.947Z", "updatedAt": "2024-08-20T13:42:36.947Z", "id": "string" }
Add Collaborator to entity
The Team has been created and members added. Now, if you want the Team to have access to an entity such as a Folder (record
), add the team as a Collaborator.
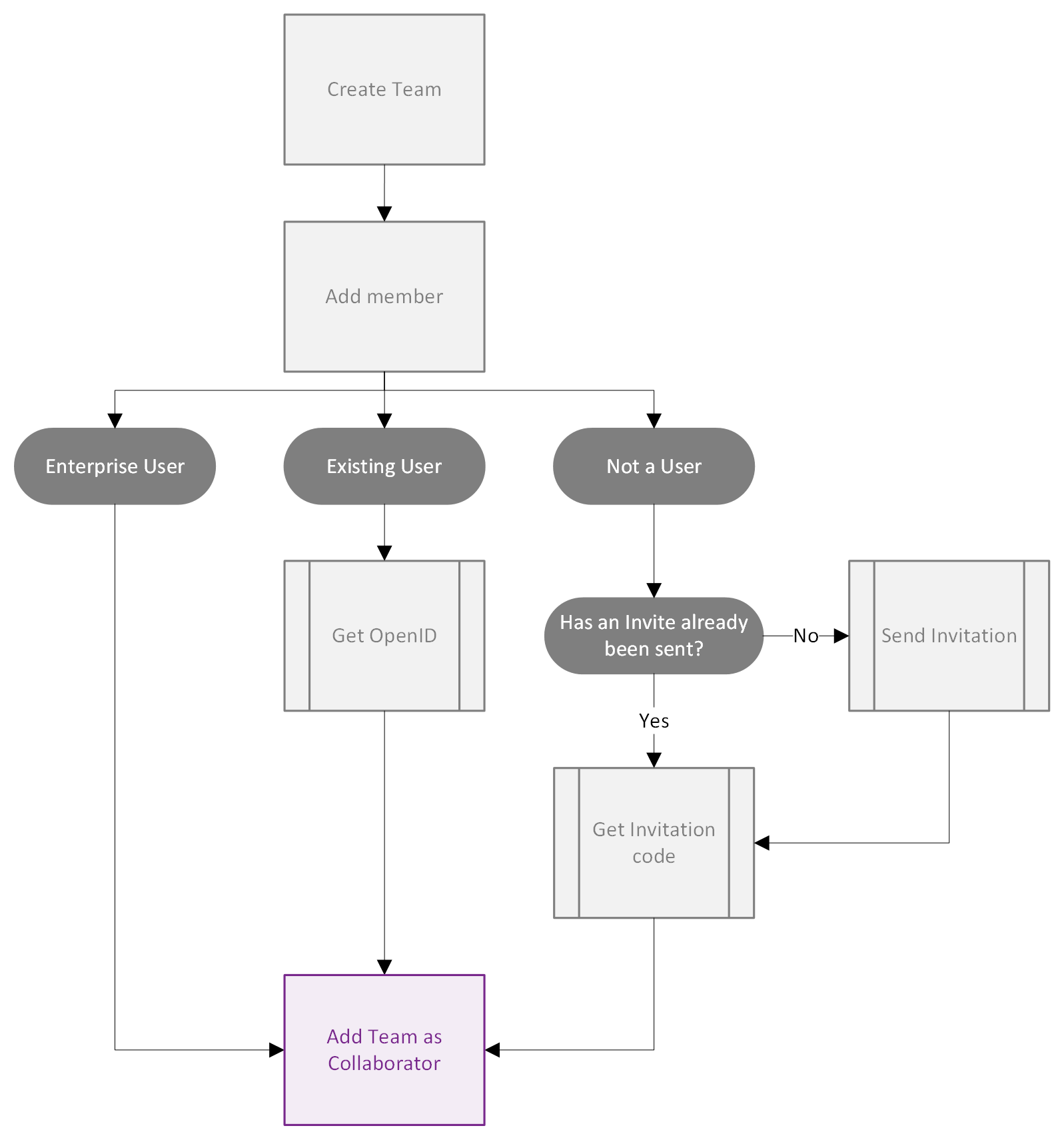
Add Team as Collaborator to Folder (
record
):
POST
/api/v1/networks/sidedrawer/sidedrawer-id/{sidedrawerId}/record/record-id/{recordId}/network
{ "recordRole": "rec_info", "contributor": { "teamId": "{teamId}", "email": null, "firstName": null }, "relation": { "personal": "other", "personalOther": "Other" }, "contributorType": "team", "expiryDate": null }
{ "active": true, "_id": "{networkId}", "teamId": "{teamId}", "sidedrawer": "{sidedrawerId}", "record": "{recordId}", "sidedrawerRole": null, "recordRole": "rec_info", "personal": "other", "region": "", "createdAt": "2024-08-21T11:58:52.021Z", "updatedAt": "2024-08-21T11:58:52.021Z", "__v": 0 }
For more information about adding Collaborators to entities, see the guide here.
Get Team members
Use this to get a list of all members of the Team.
GET
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/teams/team-id/teamId}/member
Delete Team member
You can use this endpoint to remove a Team member from the Team.
DELETE
/api/v1/tenants/tenant/tenant-id/{tenantId}/users/teams/team-id/teamId}/member
Delete Team
To delete a Team entirely, use this endpoint.
DELETE
/api/v1/tenants/tenant/tenant-id/tenantId}/users/teams/team-id/{teamId}